메인화면
메모아이콘,검색버튼,옵션메뉴,수정버튼,삭제버튼,입력버튼
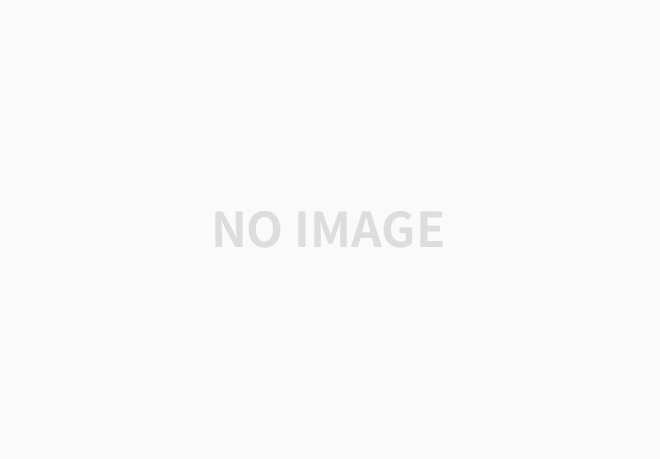
옵션메뉴를 누르면
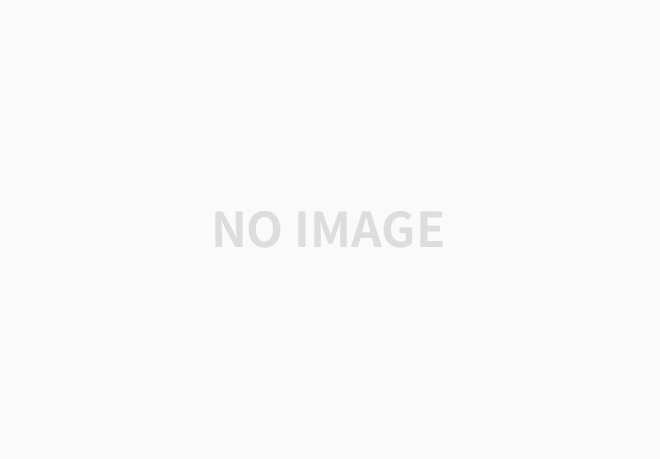
실시간 검색할수 있다.
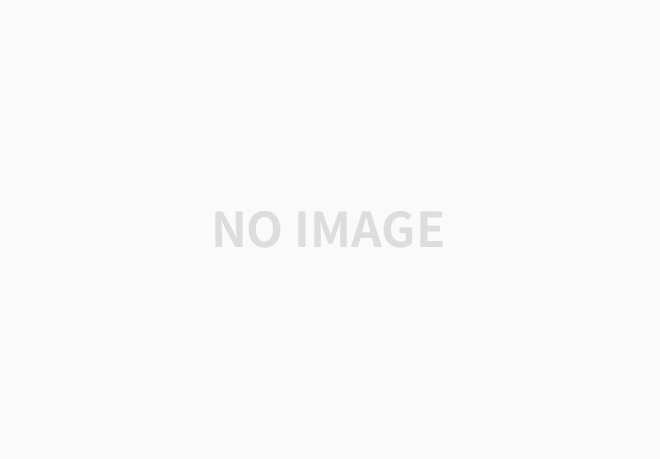
삭제할 수있다.
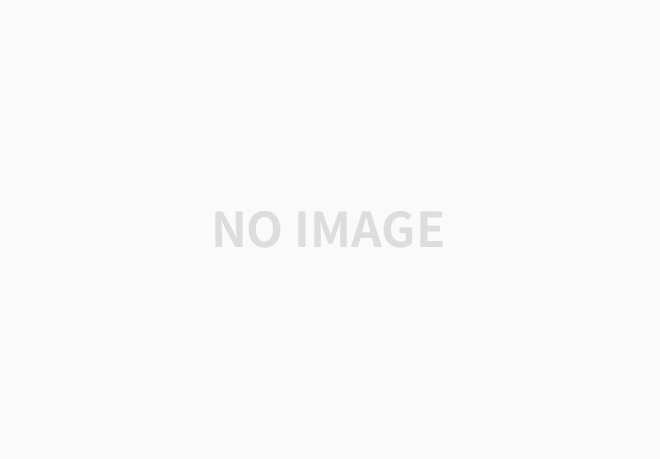
수정할수있다.
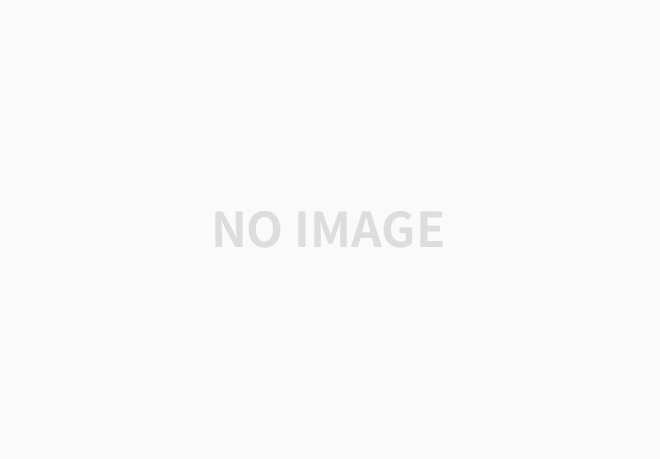
입력가능
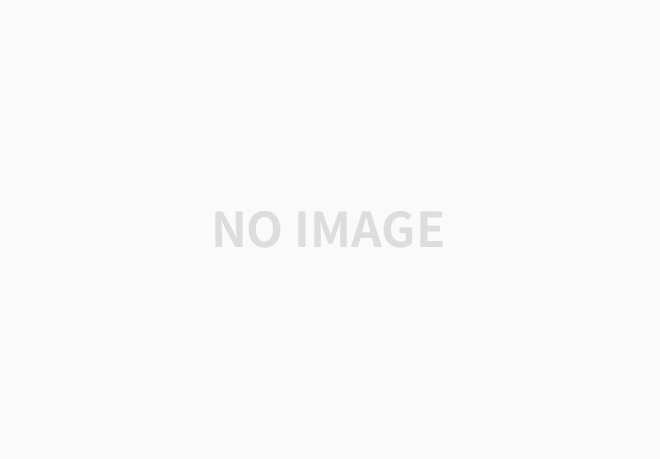
InsertActivity.java
package com.example.ex09;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.database.sqlite.SQLiteDatabase;
import android.os.Bundle;
import android.view.MenuItem;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import com.google.android.material.floatingactionbutton.FloatingActionButton;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.TimeZone;
public class InsertActivity extends AppCompatActivity {
MemoDB helper;
SQLiteDatabase db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_insert);
getSupportActionBar().setTitle("메모 입력");
//뒤로가기
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
helper=new MemoDB(this);
db=helper.getWritableDatabase();
//저장 버튼에 이벤트 달기
FloatingActionButton btnsave=findViewById(R.id.btnsave);
btnsave.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditText edtcontent=findViewById(R.id.edtcontent);
String strcontent=edtcontent.getText().toString();
//현재날짜 저장
Date now=new Date();
SimpleDateFormat sdf=new SimpleDateFormat("yyyy/MM/dd hh:mm ss");
//이상한 시간 조정
sdf.setTimeZone(TimeZone.getTimeZone("Asia/Seoul"));
String strnow=sdf.format(now);
String sql="insert into memo(content,wdate) values(";
sql += "'" + strcontent + "',";
sql += "'" + strnow + "')";
db.execSQL(sql);
Toast.makeText(InsertActivity.this, "저장되었습니다.", Toast.LENGTH_SHORT).show();
finish();
}
});
}
//뒤로가기 옵션 버튼에 이벤트주기
@Override
public boolean onOptionsItemSelected(@NonNull MenuItem item) {
switch (item.getItemId()){
case android.R.id.home:
finish();
break;
}
return super.onOptionsItemSelected(item);
}
}
activity_insert.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".InsertActivity"
android:layout_margin="10sp"
android:background="#F44336">
<EditText
android:layout_width="match_parent"
android:layout_height="match_parent"
android:hint="메모내용을 입력하세요"
android:gravity="top"
android:padding="10sp"
android:textColorHint="#ffffff"
android:id="@+id/edtcontent"/>
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/btnsave"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:clickable="true"
app:srcCompat="@drawable/ic_save"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_marginBottom="20sp"
android:layout_marginRight="20sp"
android:backgroundTint="#FFEB3B"/>
</RelativeLayout>
MainActivity.java
package com.example.ex09;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.content.DialogInterface;
import android.content.Intent;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.widget.CursorAdapter;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.SearchView;
import android.widget.TextView;
import com.google.android.material.floatingactionbutton.FloatingActionButton;
public class MainActivity extends AppCompatActivity {
MemoDB helper;
SQLiteDatabase db;
MyAdapter adapter;
Cursor cursor;
ListView list;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//헤더문구변경
getSupportActionBar().setTitle("모든 메모");
getSupportActionBar().setIcon(R.drawable.ic_memo);
//메모장 어플
getSupportActionBar().setDisplayShowHomeEnabled(true);
helper=new MemoDB(this);
db=helper.getReadableDatabase();
cursor=db.rawQuery("select * from memo order by wdate desc",null);
list=findViewById(R.id.list);
adapter=new MyAdapter(this,cursor);
list.setAdapter(adapter);
//리스터를 걸겠다.
FloatingActionButton btnwrite=findViewById(R.id.btnwrite);
btnwrite.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//activity이동
Intent intent=new Intent(MainActivity.this,InsertActivity.class);
startActivity(intent);
}
});
}
class MyAdapter extends CursorAdapter{
public MyAdapter(Context context, Cursor c) {
super(context, c);
}
@Override
public View newView(Context context, Cursor cursor, ViewGroup parent) {
//아이템 만들기
return getLayoutInflater().inflate(R.layout.item,parent,false);
}
@Override
public void bindView(View view, Context context, final Cursor cursor) {
TextView txtcontent=view.findViewById(R.id.txtcontent);
txtcontent.setText(cursor.getString(1));
TextView txtwdate=view.findViewById(R.id.txtwdate);
txtwdate.setText(cursor.getString(2));
//ListView에 item을 생성했을때
ImageView btndel=view.findViewById(R.id.btndel);
//아이디값 가져오기
final int _id=cursor.getInt(0);
//삭제버튼 이벤트 설정
btndel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//CONFRIM
AlertDialog.Builder box=new AlertDialog.Builder(MainActivity.this);
box.setMessage(_id+"을(를) 삭제하시겠습니까?");
box.setPositiveButton("확인", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
String sql="delete from memo where _id="+_id;
db.execSQL(sql);
//새로고침
onRestart();
}
});
box.setNegativeButton("닫기",null);
box.show();
}
});
ImageView btnupdate=view.findViewById(R.id.btnupdate);
btnupdate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent=new Intent(MainActivity.this,UpdateActivity.class);
intent.putExtra("_id", _id);
startActivity(intent);
}
});
}
}
//옵션메뉴 생성
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu,menu);
//검색버튼
MenuItem search=menu.findItem(R.id.search);
SearchView view=(SearchView)search.getActionView();
//query text가 변했을 때 발생
//ActionView에 리스너를 걸어준다.
view.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
//엔터를 칠때 검색
@Override
public boolean onQueryTextSubmit(String query) {
return true;
}
@Override
public boolean onQueryTextChange(String newText) {
String sql="select * from memo where content like '%" + newText + "%'";
cursor=db.rawQuery(sql,null);
adapter.changeCursor(cursor);
return false;
}
});
return super.onCreateOptionsMenu(menu);
}
//옵션메뉴를 눌렀을때 발생하는 이벤트
@Override
public boolean onOptionsItemSelected(@NonNull MenuItem item) {
switch (item.getItemId()){
case R.id.itemcontent:
cursor=db.rawQuery("select * from memo order by content",null);
break;
case R.id.itemwdate:
cursor=db.rawQuery("select * from memo order by wdate desc",null);
break;
}
//커서내용이 변경되었으므로 바뀐 커서값을 어덥터에서 바꿔줌
adapter.changeCursor(cursor);
return super.onOptionsItemSelected(item);
}
@Override
protected void onRestart() {
cursor=db.rawQuery("select * from memo order by wdate desc",null);
adapter.changeCursor(cursor);
super.onRestart();
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ListView
android:id="@+id/list"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#83F7F3F3">
</ListView>
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/btnwrite"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:clickable="true"
app:srcCompat="@drawable/ic_create"
android:backgroundTint="#FFEB3B"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_marginBottom="20sp"
android:layout_marginRight="30sp"/>
</RelativeLayout>
MemoDB.java
package com.example.ex09;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import androidx.annotation.Nullable;
//SQLiteOpenHelper 상속
public class MemoDB extends SQLiteOpenHelper {
public MemoDB(@Nullable Context context) {
super(context, "memo.db", null, 1);
}
//테이블 생성
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL("create table memo(_id integer primary key autoincrement,content text,wdate text)");
db.execSQL("insert into memo(content,wdate) values('수영장 청소','2019/11/09 06:10:30')");
db.execSQL("insert into memo(content,wdate) values('컴퓨터 조립','2019/11/19 06:10:30')");
db.execSQL("insert into memo(content,wdate) values('운동화','2019/11/04 06:10:30')");
db.execSQL("insert into memo(content,wdate) values('스트레칭','2019/11/01 06:10:30')");
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
}
}
UpdateActivity.java
package com.example.ex09;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
import android.content.DialogInterface;
import android.content.Intent;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.os.Bundle;
import android.view.MenuItem;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import com.google.android.material.floatingactionbutton.FloatingActionButton;
public class UpdateActivity extends AppCompatActivity {
int _id;
MemoDB helper;
SQLiteDatabase db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_update);
getSupportActionBar().setTitle("메모 읽기");
//뒤로가기
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
Intent intent=getIntent();
_id=intent.getIntExtra("_id",0);
helper=new MemoDB(this);
db=helper.getWritableDatabase();
Cursor cursor=db.rawQuery("select * from memo where _id="+_id, null);
if(cursor.moveToNext()){
TextView txtwdate=findViewById(R.id.txtwdate);
txtwdate.setText("작성일:"+cursor.getString(2));
TextView edtcontent=findViewById(R.id.edtcontent);
edtcontent.setText("작성내용:"+cursor.getString(1));
}
FloatingActionButton btnsave=findViewById(R.id.btnsave);
btnsave.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
AlertDialog.Builder box=new AlertDialog.Builder(UpdateActivity.this);
box.setMessage("수정하시겠습니까?");
box.setPositiveButton("확인", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
EditText edtcontent=findViewById(R.id.edtcontent);
String strcontent=edtcontent.getText().toString();
String sql="update memo set content='" + strcontent + "' ";
sql += " where _id=" +_id;
db.execSQL(sql);
finish();
}
});
box.setNegativeButton("닫기",null);
box.show();
}
});
//Toast.makeText(UpdateActivity.this,"_id="+_id,Toast.LENGTH_SHORT).show();
}
//뒤로가기 옵션 버튼에 이벤트주기
@Override
public boolean onOptionsItemSelected(@NonNull MenuItem item) {
switch (item.getItemId()){
case android.R.id.home:
finish();
break;
}
return super.onOptionsItemSelected(item);
}
}
activity_update.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".InsertActivity"
android:layout_margin="10sp"
android:background="#F44336">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/txtwdate"
android:text="작성일"
android:padding="10sp"
android:textSize="15sp"
android:textColor="#FFEB3B"/>
<EditText
android:layout_below="@id/txtwdate"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:hint="메모내용을 입력하세요"
android:gravity="top"
android:padding="10sp"
android:textColorHint="#ffffff"
android:id="@+id/edtcontent"/>
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/btnsave"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:clickable="true"
app:srcCompat="@drawable/ic_save"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_marginBottom="20sp"
android:layout_marginRight="20sp"
android:backgroundTint="#FFEB3B"/>
</RelativeLayout>
item.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#F44336"
android:layout_margin="10sp">
<TextView
android:id="@+id/txtcontent"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="여기는 내용을 출력합니다.여기는 내용을 출력합니다."
android:textColor="#FFFFFF"
android:textSize="20sp"
android:padding="10sp"
android:singleLine="true"/>
<TextView
android:id="@+id/txtwdate"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="2019/11/12 08:54:02"
android:textColor="#FFEB3B"
android:textSize="15sp"
android:padding="10sp"
android:layout_below="@id/txtcontent" />
<ImageView
android:id="@+id/btndel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_delete"
android:layout_alignParentRight="true"
/>
<ImageView
android:id="@+id/btnupdate"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_create_black_24dp"
android:layout_below="@id/txtcontent"
android:layout_alignParentRight="true"
/>
</RelativeLayout>
menu.xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item
android:id="@+id/itemcontent"
android:title="내용순 정렬"/>
<item
android:id="@+id/itemwdate"
android:title="날짜순 정렬"/>
<item
android:id="@+id/search"
android:title="검색"
android:icon="@android:drawable/ic_menu_search"
app:showAsAction="always|collapseActionView"
app:actionViewClass="android.widget.SearchView"/>
</menu>
'Android' 카테고리의 다른 글
recyclerview 사용하기 (0) | 2019.11.13 |
---|---|
Firebase를 이용한 이메일 로그인 (0) | 2019.11.12 |
캘린더를 활용한 다이어리 앱 만들기 (0) | 2019.11.11 |
주소록만들기(버튼,옵션메뉴) (0) | 2019.11.07 |
사칙연산 화면 만들기 (0) | 2019.11.07 |